PDFファイルよりテキスト抽出 iTextSharpを利用 VB.Net
NuGetより導入する。
iTextSharp
PDFファイルの表示は、WebViwer2を利用。
画面設計等
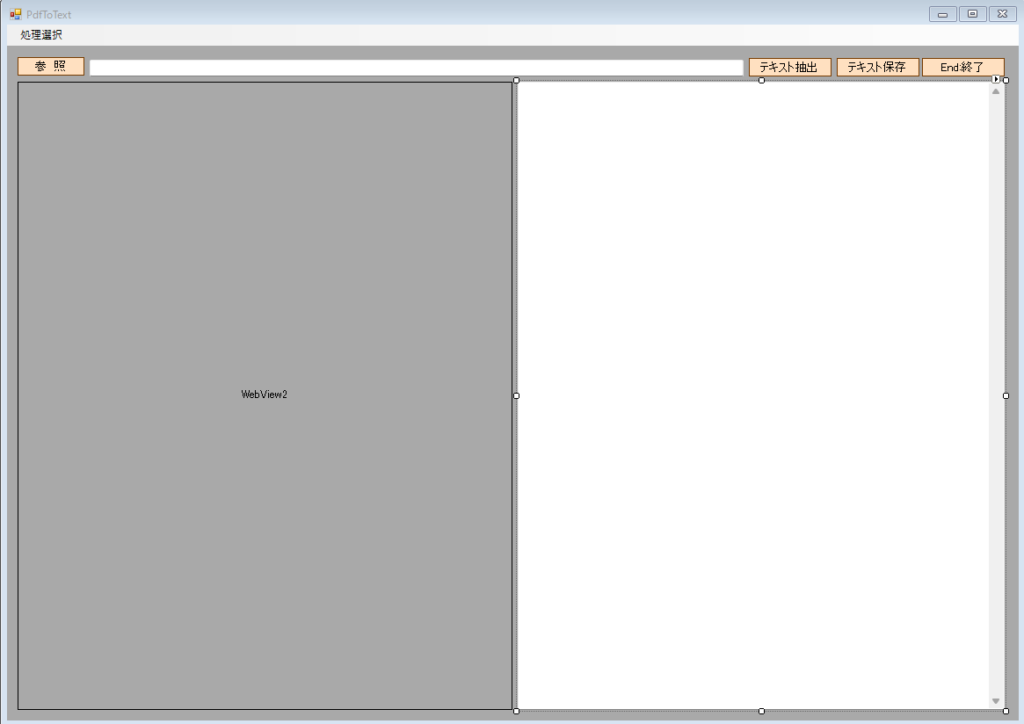
参照ボタンで、ファイル選択します。テキスト抽出ボタンで、PDFファイルからテキストを抽出します。
NuGetインストール済
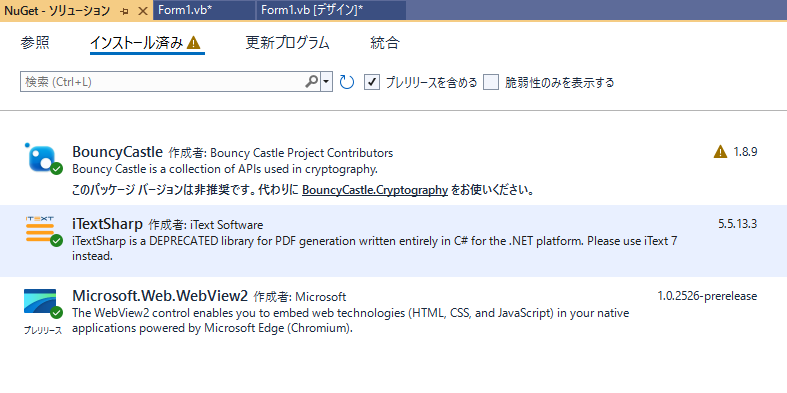
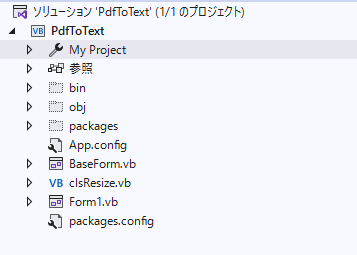
Form1.vb
Imports System.Data.OleDb
Imports System.Drawing.Drawing2D
Imports System.Drawing.Printing
Imports System.IO
Imports System.Net.WebRequestMethods
Imports System.Reflection
Imports System.Windows.Forms.VisualStyles.VisualStyleElement
Imports File = System.IO.File
Imports iTextSharp.text
Imports iTextSharp.text.pdf
Imports iTextSharp.text.pdf.parser
Imports System.Text
Imports System.Drawing
Imports System.Drawing.Imaging
Imports Image = System.Drawing.Image
Public Class Form1
Private _form_resize As clsResize
Public Sub New()
InitializeComponent()
_form_resize = New clsResize(Me)
AddHandler Me.Load, AddressOf Form1_Load
AddHandler Me.SizeChanged, AddressOf Form1_SizeChanged
End Sub
Private Sub Form1_SizeChanged(sender As Object, e As EventArgs)
_form_resize._resize()
End Sub
'-------------------------------------------------------
' フォーム初期処理
'-------------------------------------------------------
Private Sub Form1_Load(ByVal sender As System.Object,
ByVal e As System.EventArgs) Handles MyBase.Load
_form_resize._get_initial_size()
Me.KeyPreview = True
End Sub
'-------------------------------------------------------
' keyDown処理 Endボタンで終了
'-------------------------------------------------------
Private Sub Form1_KeyDown(ByVal sender As Object, ByVal e As System.Windows.Forms.KeyEventArgs) Handles MyBase.KeyDown
If e.KeyCode = Keys.End Then
End
End If
End Sub
'-------------------------------------------------------
' keyPress処理 Enter = tab
'-------------------------------------------------------
Private Sub Form1_KeyPress(ByVal sender As Object, ByVal e As System.Windows.Forms.KeyPressEventArgs) Handles MyBase.KeyPress
If e.KeyChar = Chr(13) Then
SendKeys.Send("{TAB}")
e.Handled = True
End If
End Sub
'-------------------------------------------------------
' Textbox1 KeyDown処理
'-------------------------------------------------------
Private Sub TextBox1_KeyDown(ByVal sender As Object, ByVal e As System.Windows.Forms.KeyEventArgs) Handles TextBox1.KeyDown
If e.KeyCode = Keys.Enter And TextBox1.Text IsNot String.Empty Then
Me.WebView21.Source = New Uri(TextBox1.Text)
End If
End Sub
'-------------------------------------------------------
' 参照ボタン処理
'-------------------------------------------------------
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
Dim ofd As OpenFileDialog = New OpenFileDialog()
ofd.InitialDirectory = System.Environment.CurrentDirectory
ofd.Filter = "PDFファイル(*.pdf)|*.pdf"
If ofd.ShowDialog() = DialogResult.OK Then
WebView21.Source = New Uri(ofd.FileName)
TextBox1.Text = ofd.FileName
End If
End Sub
'-------------------------------------------------------
' 終了メニュー処理
'-------------------------------------------------------
Private Sub 終了ToolStripMenuItem_Click(sender As Object, e As EventArgs) Handles 終了ToolStripMenuItem.Click
End
End Sub
'-------------------------------------------------------
' 終了ボタン処理
'-------------------------------------------------------
Private Sub Button2_Click(sender As Object, e As EventArgs) Handles Button2.Click
End
End Sub
'テキスト抽出
Private Sub Button5_Click(sender As Object, e As EventArgs) Handles Button5.Click
Try
Dim text As StringBuilder = New StringBuilder()
Using pr As PdfReader = New PdfReader(TextBox1.Text)
For page As Integer = 1 To pr.NumberOfPages
Dim pageText As String = PdfTextExtractor.GetTextFromPage(pr, page, New LocationTextExtractionStrategy())
Dim lines() As String = pageText.Split(vbLf)
For i As Integer = 0 To lines.Length - 1
text.Append(lines(i) + vbCrLf)
Next
Next
pr.Close()
End Using
TextBox2.Text = text.ToString()
Catch ex As Exception
Throw ex
End Try
End Sub
'テキスト保存
Private Sub Button6_Click(sender As Object, e As EventArgs) Handles Button6.Click
'SaveFileDialogクラスのインスタンスを作成
Dim sfd As New SaveFileDialog()
'はじめのファイル名を指定する
'はじめに「ファイル名」で表示される文字列を指定する
sfd.FileName = "新しいファイル.txt"
'はじめに表示されるフォルダを指定する
'指定しない(空の文字列)の時は、現在のディレクトリが表示される
sfd.InitialDirectory = System.Environment.CurrentDirectory
'[ファイルの種類]に表示される選択肢を指定する
sfd.Filter = "テキストファイル(*.txt)|*.txt|すべてのファイル(*.*)|*.*"
'[ファイルの種類]ではじめに選択されるものを指定する
'2番目の「すべてのファイル」が選択されているようにする
sfd.FilterIndex = 1
'タイトルを設定する
sfd.Title = "保存先のファイルを選択してください"
'ダイアログボックスを閉じる前に現在のディレクトリを復元するようにする
sfd.RestoreDirectory = True
'既に存在するファイル名を指定したとき警告する
'デフォルトでTrueなので指定する必要はない
sfd.OverwritePrompt = True
'存在しないパスが指定されたとき警告を表示する
'デフォルトでTrueなので指定する必要はない
sfd.CheckPathExists = True
'ダイアログを表示する
If sfd.ShowDialog() = DialogResult.OK Then
'OKボタンがクリックされたとき、選択されたファイル名を表示する
Console.WriteLine(sfd.FileName)
'テキストファイルを上書きで保存する
Dim sw As New System.IO.StreamWriter(sfd.FileName, False,
System.Text.Encoding.Default)
'書込み
sw.Write(TextBox2.Text)
sw.Close()
End If
End Sub
End Class
clsResize.cs
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Text
Imports System.Windows.Forms
Public Class clsResize
Private _arr_control_storage As List(Of System.Drawing.Rectangle) = New List(Of System.Drawing.Rectangle)()
Private showRowHeader As Boolean = False
Private FontTable As Dictionary(Of String, Single)
Private ControlTable As Dictionary(Of String, System.Drawing.Rectangle)
Public Sub New(ByVal _form_ As Form)
form = _form_
_formSize = _form_.ClientSize
_fontsize = _form_.Font.Size
Dim _controls = _get_all_controls(form)
FontTable = New Dictionary(Of String, Single)()
ControlTable = New Dictionary(Of String, System.Drawing.Rectangle)()
For Each control As Control In _controls
FontTable.Add(control.Name, control.Font.Size)
ControlTable.Add(control.Name, control.Bounds)
Next
End Sub
Private _fontsize As Single
Private _formSize As System.Drawing.SizeF
Private form As Form
Public Sub _get_initial_size()
Dim _controls = _get_all_controls(form)
For Each control As Control In _controls
_arr_control_storage.Add(control.Bounds)
If control.[GetType]() = GetType(DataGridView) Then _dgv_Column_Adjust((CType(control, DataGridView)), showRowHeader)
Next
End Sub
Public Sub _resize()
Dim _form_ratio_width As Double = CDbl(form.ClientSize.Width) / CDbl(_formSize.Width)
Dim _form_ratio_height As Double = CDbl(form.ClientSize.Height) / CDbl(_formSize.Height)
Dim _controls = _get_all_controls(form)
Dim _pos As Integer = -1
For Each control As Control In _controls
Me._fontsize = FontTable(control.Name)
_pos += 1
Dim _controlSize As System.Drawing.Size = New System.Drawing.Size(CInt((_arr_control_storage(_pos).Width * _form_ratio_width)), CInt((_arr_control_storage(_pos).Height * _form_ratio_height)))
Dim _controlposition As System.Drawing.Point = New System.Drawing.Point(CInt((_arr_control_storage(_pos).X * _form_ratio_width)), CInt((_arr_control_storage(_pos).Y * _form_ratio_height)))
control.Bounds = New System.Drawing.Rectangle(_controlposition, _controlSize)
If control.[GetType]() = GetType(DataGridView) Then _dgv_Column_Adjust((CType(control, DataGridView)), showRowHeader)
control.Font = New System.Drawing.Font(form.Font.FontFamily, CSng((((Convert.ToDouble(_fontsize) * _form_ratio_width) / 2) + ((Convert.ToDouble(_fontsize) * _form_ratio_height) / 2))))
Next
End Sub
Private Sub _dgv_Column_Adjust(ByVal dgv As DataGridView, ByVal _showRowHeader As Boolean)
Dim intRowHeader As Integer = 0
Const Hscrollbarwidth As Integer = 5
If _showRowHeader Then
intRowHeader = dgv.RowHeadersWidth
Else
dgv.RowHeadersVisible = False
End If
For i As Integer = 0 To dgv.ColumnCount - 1
If dgv.Dock = DockStyle.Fill Then
dgv.Columns(i).Width = ((dgv.Width - intRowHeader) / dgv.ColumnCount)
Else
dgv.Columns(i).Width = ((dgv.Width - intRowHeader - Hscrollbarwidth) / dgv.ColumnCount)
End If
Next
End Sub
Private Shared Function _get_all_controls(ByVal c As Control) As IEnumerable(Of Control)
Return c.Controls.Cast(Of Control)().SelectMany(Function(item) _get_all_controls(item)).Concat(c.Controls.Cast(Of Control)()).Where(Function(control) control.Name <> String.Empty)
End Function
End Class
BaseForm.vb
Public Class BaseForm
Private resizeHelper As clsResize
Public Sub New()
InitializeComponent()
OnLoad(EventArgs.Empty)
End Sub
Protected Overrides Sub OnLoad(e As EventArgs)
If Not DesignMode Then
MyBase.OnLoad(e)
resizeHelper = New clsResize(Me)
resizeHelper._get_initial_size()
End If
End Sub
Protected Overrides Sub OnResize(e As EventArgs)
If Not DesignMode Then
If resizeHelper Is Nothing Then
resizeHelper = New clsResize(Me)
resizeHelper._get_initial_size()
Else
resizeHelper._resize()
End If
End If
End Sub
End Class
Form1.Designer.vb
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()>
Partial Class Form1
'Inherits System.Windows.Forms.Form
Inherits BaseForm
'フォームがコンポーネントの一覧をクリーンアップするために dispose をオーバーライドします。
<System.Diagnostics.DebuggerNonUserCode()>
Protected Overrides Sub Dispose(ByVal disposing As Boolean)
Try
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
Finally
MyBase.Dispose(disposing)
End Try
End Sub
'Windows フォーム デザイナーで必要です。
Private components As System.ComponentModel.IContainer
実行画面
PDFファイルを選択し、テキスト抽出しました。
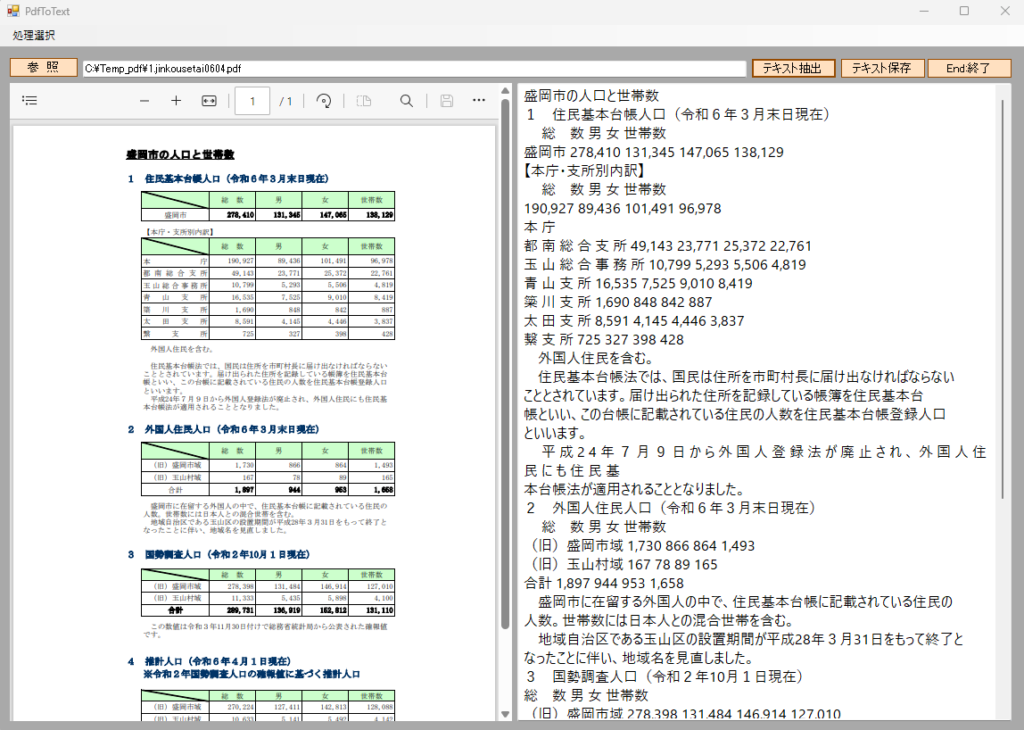