SwingでiTextを利用して一覧表を作成
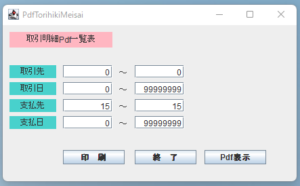
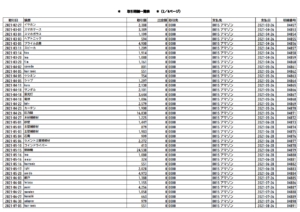
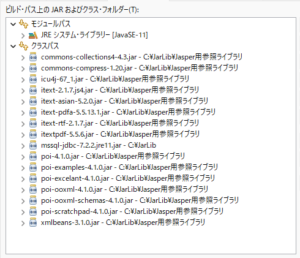
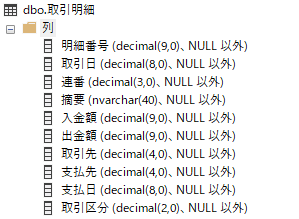
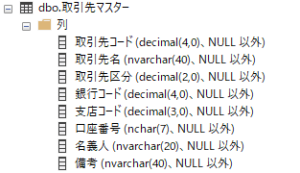
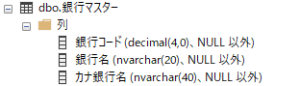
import java.awt.Color;
import java.awt.EventQueue;
import java.awt.Label;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.FocusAdapter;
import java.awt.event.FocusEvent;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.SwingConstants;
import javax.swing.border.EmptyBorder;
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.Element;
import com.itextpdf.text.Font;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.Phrase;
import com.itextpdf.text.pdf.BaseFont;
import com.itextpdf.text.pdf.PdfPCell;
import com.itextpdf.text.pdf.PdfPTable;
import com.itextpdf.text.pdf.PdfPageEventHelper;
import com.itextpdf.text.pdf.PdfWriter;
public class PdfTorihikiMeisai extends JFrame {
private JPanel contentPane;
private JTextField textField;
private JTextField textField_1;
private ResultSet rs;
private JTextField textField_2;
private JTextField textField_3;
private JTextField textField_4;
private JTextField textField_5;
private JTextField textField_6;
private JTextField textField_7;
private int sttcode=0;
private int endcode=0;
private int sttcode2=0;
private int endcode2=0;
private int sttcode3=0;
private int endcode3=0;
private int sttcode4=0;
private int endcode4=0;
private String PathName =”c:\\sample\\pdftorihikimeisai.pdf”;
private String table[][];
//レコード数
private int num_line;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
PdfTorihikiMeisai frame = new PdfTorihikiMeisai();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public PdfTorihikiMeisai() {
setTitle(“PdfTorihikiMeisai”);
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
setBounds(100, 100, 450, 269);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
Label label = new Label(“取引明細Pdf一覧表”);
label.setBackground(new Color(255, 182, 193));
label.setAlignment(Label.CENTER);
label.setBounds(10, 10, 152, 23);
contentPane.add(label);
Label label_1 = new Label(“取引先”);
label_1.setBackground(new Color(72, 209, 204));
label_1.setAlignment(Label.CENTER);
label_1.setBounds(10, 59, 69, 19);
contentPane.add(label_1);
textField = new JTextField();
textField.setBounds(89, 59, 73, 19);
textField.setDocument( new TextTypeLimit(TextType.NUMERIC,4));
textField.setHorizontalAlignment(SwingConstants.RIGHT);
textField.setText(“0”);
textField.addFocusListener(new FocusAdapter() {
@Override public void focusGained(FocusEvent e) {
((JTextField) e.getComponent()).selectAll();
}
});
textField.addKeyListener(new KeyListener() {
@Override
public void keyTyped(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
@Override
public void keyPressed(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
if (e.getKeyCode() == KeyEvent.VK_ENTER){
textField_1.requestFocus();
}
}
@Override
public void keyReleased(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
});
contentPane.add(textField);
Label label_2 = new Label(“~”);
label_2.setAlignment(Label.CENTER);
label_2.setBounds(168, 59, 21, 23);
contentPane.add(label_2);
textField_1 = new JTextField();
textField_1.setBounds(195, 59, 73, 19);
textField_1.setDocument( new TextTypeLimit(TextType.NUMERIC,4));
textField_1.setHorizontalAlignment(SwingConstants.RIGHT);
textField_1.setText(“9999”);
textField_1.addFocusListener(new FocusAdapter() {
@Override public void focusGained(FocusEvent e) {
((JTextField) e.getComponent()).selectAll();
}
});
textField_1.addKeyListener(new KeyListener() {
@Override
public void keyTyped(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
@Override
public void keyPressed(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
if (e.getKeyCode() == KeyEvent.VK_ENTER){
textField_2.requestFocus();
}
}
@Override
public void keyReleased(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
});
contentPane.add(textField_1);
Label label_3 = new Label(“取引日”);
label_3.setBackground(new Color(72, 209, 204));
label_3.setAlignment(Label.CENTER);
label_3.setBounds(10, 84, 69, 19);
contentPane.add(label_3);
textField_2 = new JTextField();
textField_2.setHorizontalAlignment(SwingConstants.RIGHT);
textField_2.setBounds(89, 84, 73, 19);
textField_2.setDocument( new TextTypeLimit(TextType.NUMERIC,8));
textField_2.setText(“0”);
textField_2.addFocusListener(new FocusAdapter() {
@Override public void focusGained(FocusEvent e) {
((JTextField) e.getComponent()).selectAll();
}
});
textField_2.addKeyListener(new KeyListener() {
@Override
public void keyTyped(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
@Override
public void keyPressed(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
if (e.getKeyCode() == KeyEvent.VK_ENTER){
textField_3.requestFocus();
}
}
@Override
public void keyReleased(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
});
contentPane.add(textField_2);
textField_3 = new JTextField();
textField_3.setHorizontalAlignment(SwingConstants.RIGHT);
textField_3.setBounds(195, 84, 73, 19);
textField_3.setDocument( new TextTypeLimit(TextType.NUMERIC,8));
textField_3.setText(“99999999”);
textField_3.addFocusListener(new FocusAdapter() {
@Override public void focusGained(FocusEvent e) {
((JTextField) e.getComponent()).selectAll();
}
});
textField_3.addKeyListener(new KeyListener() {
@Override
public void keyTyped(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
@Override
public void keyPressed(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
if (e.getKeyCode() == KeyEvent.VK_ENTER){
textField_4.requestFocus();
}
}
@Override
public void keyReleased(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
});
contentPane.add(textField_3);
Label label_4 = new Label(“支払先”);
label_4.setBackground(new Color(72, 209, 204));
label_4.setAlignment(Label.CENTER);
label_4.setBounds(10, 109, 69, 19);
contentPane.add(label_4);
Label label_5 = new Label(“~”);
label_5.setAlignment(Label.CENTER);
label_5.setBounds(168, 84, 21, 23);
contentPane.add(label_5);
textField_4 = new JTextField();
textField_4.setHorizontalAlignment(SwingConstants.RIGHT);
textField_4.setBounds(89, 109, 73, 19);
textField_4.setDocument( new TextTypeLimit(TextType.NUMERIC,4));
textField_4.setText(“0”);
textField_4.addFocusListener(new FocusAdapter() {
@Override public void focusGained(FocusEvent e) {
((JTextField) e.getComponent()).selectAll();
}
});
textField_4.addKeyListener(new KeyListener() {
@Override
public void keyTyped(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
@Override
public void keyPressed(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
if (e.getKeyCode() == KeyEvent.VK_ENTER){
textField_5.requestFocus();
}
}
@Override
public void keyReleased(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
});
contentPane.add(textField_4);
textField_5 = new JTextField();
textField_5.setHorizontalAlignment(SwingConstants.RIGHT);
textField_5.setBounds(195, 109, 73, 19);
textField_5.setDocument( new TextTypeLimit(TextType.NUMERIC,4));
textField_5.setText(“9999”);
textField_5.addFocusListener(new FocusAdapter() {
@Override public void focusGained(FocusEvent e) {
((JTextField) e.getComponent()).selectAll();
}
});
textField_5.addKeyListener(new KeyListener() {
@Override
public void keyTyped(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
@Override
public void keyPressed(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
if (e.getKeyCode() == KeyEvent.VK_ENTER){
textField_6.requestFocus();
}
}
@Override
public void keyReleased(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
});
contentPane.add(textField_5);
Label label_6 = new Label(“~”);
label_6.setAlignment(Label.CENTER);
label_6.setBounds(168, 109, 21, 23);
contentPane.add(label_6);
Label label_7 = new Label(“支払日”);
label_7.setBackground(new Color(72, 209, 204));
label_7.setAlignment(Label.CENTER);
label_7.setBounds(10, 134, 69, 19);
contentPane.add(label_7);
textField_6 = new JTextField();
textField_6.setHorizontalAlignment(SwingConstants.RIGHT);
textField_6.setBounds(89, 134, 73, 19);
textField_6.setDocument( new TextTypeLimit(TextType.NUMERIC,8));
textField_6.setText(“0”);
textField_6.addFocusListener(new FocusAdapter() {
@Override public void focusGained(FocusEvent e) {
((JTextField) e.getComponent()).selectAll();
}
});
textField_6.addKeyListener(new KeyListener() {
@Override
public void keyTyped(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
@Override
public void keyPressed(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
if (e.getKeyCode() == KeyEvent.VK_ENTER){
textField_7.requestFocus();
}
}
@Override
public void keyReleased(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
});
contentPane.add(textField_6);
textField_7 = new JTextField();
textField_7.setHorizontalAlignment(SwingConstants.RIGHT);
textField_7.setBounds(195, 134, 73, 19);
textField_7.setDocument( new TextTypeLimit(TextType.NUMERIC,8));
textField_7.setText(“99999999”);
textField_7.addFocusListener(new FocusAdapter() {
@Override public void focusGained(FocusEvent e) {
((JTextField) e.getComponent()).selectAll();
}
});
textField_7.addKeyListener(new KeyListener() {
@Override
public void keyTyped(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
@Override
public void keyPressed(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
if (e.getKeyCode() == KeyEvent.VK_ENTER){
textField.requestFocus();
}
}
@Override
public void keyReleased(KeyEvent e) {
// TODO 自動生成されたメソッド・スタブ
}
});
contentPane.add(textField_7);
Label label_8 = new Label(“~”);
label_8.setAlignment(Label.CENTER);
label_8.setBounds(168, 134, 21, 23);
contentPane.add(label_8);
JButton button = new JButton(“印 刷”);
button.setBounds(89, 184, 91, 21);
button.addActionListener(new ActionListener() {
@SuppressWarnings(“static-access”)
public void actionPerformed(ActionEvent e) {
try {
sttcode =Integer.parseInt(textField.getText());
endcode =Integer.parseInt(textField_1.getText());
sttcode2 =Integer.parseInt(textField_2.getText());
endcode2 =Integer.parseInt(textField_3.getText());
sttcode3 =Integer.parseInt(textField_4.getText());
endcode3 =Integer.parseInt(textField_5.getText());
sttcode4 =Integer.parseInt(textField_6.getText());
endcode4 =Integer.parseInt(textField_7.getText());
} catch (Exception e2) {
JOptionPane.showMessageDialog(button,”範囲指定が間違っています。”,
“インフォメーション”, JOptionPane.INFORMATION_MESSAGE);
textField.requestFocus();
return;
}
if (sttcode > endcode) {
JOptionPane.showMessageDialog(button,”取引先範囲指定が間違っています。”,
“インフォメーション”, JOptionPane.INFORMATION_MESSAGE);
textField.requestFocus();
return;
}
if (sttcode2 > endcode2) {
JOptionPane.showMessageDialog(button,”取引日範囲指定が間違っています。”,
“インフォメーション”, JOptionPane.INFORMATION_MESSAGE);
textField.requestFocus();
return;
}
if (sttcode3 > endcode3) {
JOptionPane.showMessageDialog(button,”支払先範囲指定が間違っています。”,
“インフォメーション”, JOptionPane.INFORMATION_MESSAGE);
textField.requestFocus();
return;
}
if (sttcode4 > endcode4) {
JOptionPane.showMessageDialog(button,”支払日範囲指定が間違っています。”,
“インフォメーション”, JOptionPane.INFORMATION_MESSAGE);
textField.requestFocus();
return;
}
SetTable();
CreatePdf createPdf = new CreatePdf();
createPdf.execute();
try {
if ((new File(PathName)).exists()) {
Process p = Runtime
.getRuntime()
.exec(“rundll32 url.dll,FileProtocolHandler ” + PathName);
p.waitFor();
} else {
JOptionPane.showMessageDialog(contentPane, “File is not exists”,
“インフォメーション”, JOptionPane.INFORMATION_MESSAGE);
//System.out.println(“File is not exists”);
}
//JOptionPane.showMessageDialog(contentPane, “Done”,
// “インフォメーション”, JOptionPane.INFORMATION_MESSAGE);
//System.out.println(“Done”);
} catch (Exception ex) {
ex.printStackTrace();
}
}
});
contentPane.add(button);
JButton button_1 = new JButton(“終 了”);
button_1.setBounds(195, 184, 91, 21);
button_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
//System.out.println(“ENDキーが押された”);
//System.exit(0);
dispose();
}
});
contentPane.add(button_1);
JButton btnPdf = new JButton(“Pdf表示”);
btnPdf.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
if ((new File(PathName)).exists()) {
Process p = Runtime
.getRuntime()
.exec(“rundll32 url.dll,FileProtocolHandler ” + PathName);
p.waitFor();
} else {
JOptionPane.showMessageDialog(contentPane, “File is not exists”,
“インフォメーション”, JOptionPane.INFORMATION_MESSAGE);
//System.out.println(“File is not exists”);
}
//JOptionPane.showMessageDialog(contentPane, “Done”,
// “インフォメーション”, JOptionPane.INFORMATION_MESSAGE);
//System.out.println(“Done”);
} catch (Exception ex) {
ex.printStackTrace();
}
}
});
btnPdf.setBounds(298, 184, 91, 21);
contentPane.add(btnPdf);
}
@SuppressWarnings(“static-access”)
private void SetTable() {
Connect Con = new Connect();
Con.open();
String Str =””;
Str = “SELECT 取引明細.*,coalesce(取引先.取引先名,”) as torihikisakimei,coalesce(支払先.取引先名,”) as shiharaisakimei FROM 取引明細”;
Str += ” left outer join 取引先マスター as 取引先 on 取引先.取引先コード = 取引明細.取引先”;
Str += ” left outer join 取引先マスター as 支払先 on 支払先.取引先コード = 取引明細.支払先”;
Str += ” Where 取引先>=” + sttcode;
Str += ” and 取引先<=” + endcode;
Str += ” and 取引日>=” + sttcode2;
Str += ” and 取引日<=” + endcode2;
Str += ” and 支払先>=” + sttcode3;
Str += ” and 支払先<=” + endcode3;
Str += ” and 支払日>=” + sttcode4;
Str += ” and 支払日<=” + endcode4;
Str += ” order By 取引日 “;
String StrCount =””;
StrCount = “SELECT coalesce(count(*),0) as 件数 FROM 取引明細”;
StrCount += ” Where 取引先>=” + sttcode;
StrCount += ” and 取引先<=” + endcode;
StrCount += ” and 取引日>=” + sttcode2;
StrCount += ” and 取引日<=” + endcode2;
StrCount += ” and 支払先>=” + sttcode3;
StrCount += ” and 支払先<=” + endcode3;
StrCount += ” and 支払日>=” + sttcode4;
StrCount += ” and 支払日<=” + endcode4;
try {
//データ取得
int Count=0;
rs = Con.stmt.executeQuery(StrCount);
while (rs.next()) {
Count=rs.getInt(“件数”);
}
rs.close();
//System.out.println(Count);;
table = new String[Count][10];
for(String[] x: table){
java.util.Arrays.fill(x,””);
}
num_line=0;
rs = Con.stmt.executeQuery(Str);
while (rs.next()) {
String F01 = null;
table[num_line][0] = rs.getString(“取引日”).substring(0,4) +”-” + rs.getString(“取引日”).substring(4,6) + “-” + rs.getString(“取引日”).substring(6,8);
String F02 =rs.getString(“摘要”);
table[num_line][1] =rs.getString(“摘要”);
String F03=null;
table[num_line][2] = F03.format(“%,d”,rs.getInt(“入金額”));
String F04=null;
table[num_line][3] = F04.format(“%,d”,rs.getInt(“出金額”));
String F05 = null;
table[num_line][4] = F05.format(“%04d”,rs.getInt(“取引先”));
String F06= rs.getString(“torihikisakimei”);
table[num_line][5] = F06;
String F07 = null;
table[num_line][6] = F07.format(“%04d”,rs.getInt(“支払先”));
if (rs.getInt(“支払先”)==0) {
table[num_line][6] =””;
}
String F08=rs.getString(“shiharaisakimei”);
table[num_line][7] = F08;
String F09 = null;
if (rs.getInt(“支払日”) < 10000000) {
table[num_line][8] =””;
} else {
table[num_line][8] = rs.getString(“支払日”).substring(0,4) +”-” + rs.getString(“支払日”).substring(4,6) + “-” + rs.getString(“支払日”).substring(6,8);
}
String F10= null;
table[num_line][9]= F10.format(“%05d”,rs.getInt(“明細番号”));
num_line = num_line + 1;
}
rs.close();
}catch(SQLException ex) {
JOptionPane.showMessageDialog(contentPane, “選択に失敗しました。”,
“インフォメーション”, JOptionPane.INFORMATION_MESSAGE);
if(rs!=null)
try{rs.close();}catch(Exception e2){ex.printStackTrace();}
ex.printStackTrace();
return;
}
Con.close();
}
class CreatePdf extends PdfPageEventHelper {
//———————————–
// フォント
//———————————–
/** 平成明朝体 */
private static final String MINCHO = “HeiseiMin-W3”;
/** 平成角ゴシック体. */
private static final String GOTHIC = “HeiseiKakuGo-W5”;
/** 横書き指定 */
private static final String HORIZONTAL = “UniJIS-UCS2-H”;
/** 縦書き指定 */
private static final String VERTICAL = “UniJIS-UCS2-V”;
/** 横書き指定 & 英数を半角幅で印字 */
private static final String HORIZONTAL_HW = “UniJIS-UCS2-HW-H”;
/** 縦書き指定 & 英数を半角幅で印字 */
private static final String VERTICAL_HW = “UniJIS-UCS2-HW-V”;
/** ベースフォント 明朝体 */
private BaseFont mincho = null;
/** ベースフォント ゴシック体 */
private BaseFont gothic = null;
private Font mincho15 = null;
/** 全ページ数 */
private int pageCount = 0;
/** 1ページリスト表示行数 */
private final int LIST_ROW = 40;
/** 明細一覧テーブルの列幅定義 */
float widthC[] = { 8, 30, 11, 11, 20, 20,10 };
public void execute() {
//文書オブジェクトを生成 rotate指定で横書き
Document doc = new Document(PageSize.A4.rotate(), 10, 10, 50, 10);
//出力先(アウトプットストリーム)の生成
FileOutputStream fos = null;
try {
fos = new FileOutputStream(PathName);
} catch (FileNotFoundException e2) {
// TODO 自動生成された catch ブロック
e2.printStackTrace();
}
//アウトプットストリームをPDFWriterに設定
PdfWriter pdfwriter = null;
try {
pdfwriter = PdfWriter.getInstance(doc, fos);
//ページ制御
pdfwriter.setPageEvent(this);
} catch (DocumentException e2) {
// TODO 自動生成された catch ブロック
e2.printStackTrace();
}
//フォントの設定
Font font = null;
Font font8 = null;
Font fontB =null;
Font fontU =null;
try {
font = new Font(BaseFont.createFont(“HeiseiKakuGo-W5”,
“UniJIS-UCS2-H”,BaseFont.NOT_EMBEDDED),9);
font8 = new Font(BaseFont.createFont(“HeiseiKakuGo-W5”,
“UniJIS-UCS2-H”,BaseFont.NOT_EMBEDDED),8);
fontB = new Font(BaseFont.createFont(“HeiseiKakuGo-W5”,
“UniJIS-UCS2-H”,BaseFont.NOT_EMBEDDED),9, Font.BOLD);
fontU= new Font(BaseFont.createFont(“HeiseiKakuGo-W5”,
“UniJIS-UCS2-H”,BaseFont.NOT_EMBEDDED),9, Font.UNDERLINE);
} catch (DocumentException | IOException e2) {
// TODO 自動生成された catch ブロック
e2.printStackTrace();
}
//文章オブジェクト オープン
doc.open();
try {
Paragraph p = new Paragraph();
int x = 0;
//明細行の表を作成(7列)
PdfPTable pdfPTable = new PdfPTable(8);
pdfPTable.setHorizontalAlignment(Element.ALIGN_CENTER);
/** テーブルの列幅定義 */
float width1[] = { 8, 40, 8, 8, 17, 17,8,8 };
pdfPTable.setWidths(width1);
pdfPTable.setWidthPercentage(100f);
// ページ数の算出
pageCount = num_line%LIST_ROW == 0 ?
num_line/LIST_ROW : num_line/LIST_ROW +1 ;
for (int i = 0; i < num_line; i++) {
if ((i == 0 || i % LIST_ROW == 0)) {
PdfPCell cell1_01 = new PdfPCell(new Paragraph(“取引日”, font));
cell1_01.setHorizontalAlignment(Element.ALIGN_CENTER);
PdfPCell cell1_02 = new PdfPCell(new Paragraph(“摘要”, font));
cell1_02.setHorizontalAlignment(Element.ALIGN_LEFT);
PdfPCell cell1_03 = new PdfPCell(new Paragraph(“取引額”, font));
cell1_03.setHorizontalAlignment(Element.ALIGN_RIGHT);
PdfPCell cell1_04 = new PdfPCell(new Paragraph(“出金額”, font));
cell1_04.setHorizontalAlignment(Element.ALIGN_RIGHT);
PdfPCell cell1_05 = new PdfPCell(new Paragraph(“取引先”, font));
cell1_05.setHorizontalAlignment(Element.ALIGN_LEFT);
PdfPCell cell1_06 = new PdfPCell(new Paragraph(“支払先”, font));
cell1_06.setHorizontalAlignment(Element.ALIGN_LEFT);
PdfPCell cell1_07 = new PdfPCell(new Paragraph(“支払日”, font));
cell1_07.setHorizontalAlignment(Element.ALIGN_CENTER);
PdfPCell cell1_08 = new PdfPCell(new Paragraph(“明細番号”, font));
cell1_08.setHorizontalAlignment(Element.ALIGN_RIGHT);
pdfPTable.addCell(cell1_01);
pdfPTable.addCell(cell1_02);
pdfPTable.addCell(cell1_03);
pdfPTable.addCell(cell1_04);
pdfPTable.addCell(cell1_05);
pdfPTable.addCell(cell1_06);
pdfPTable.addCell(cell1_07);
pdfPTable.addCell(cell1_08);
}
PdfPCell cell1_01 = new PdfPCell(new Paragraph(table[i][0], font));
cell1_01.setHorizontalAlignment(Element.ALIGN_CENTER);
PdfPCell cell1_02 = new PdfPCell(new Paragraph(table[i][1], font8));
cell1_02.setHorizontalAlignment(Element.ALIGN_LEFT);
PdfPCell cell1_03 = new PdfPCell(new Paragraph(table[i][2], font));
cell1_03.setHorizontalAlignment(Element.ALIGN_RIGHT);
PdfPCell cell1_04 = new PdfPCell(new Paragraph(table[i][3], font));
cell1_04.setHorizontalAlignment(Element.ALIGN_RIGHT);
PdfPCell cell1_05 = new PdfPCell(new Paragraph(table[i][4] + ” ” + table[i][5], font));
cell1_05.setHorizontalAlignment(Element.ALIGN_LEFT);
PdfPCell cell1_06 = new PdfPCell(new Paragraph(table[i][6] + ” ” + table[i][7], font));
cell1_06.setHorizontalAlignment(Element.ALIGN_LEFT);
PdfPCell cell1_07 = new PdfPCell(new Paragraph(table[i][8], font));
cell1_07.setHorizontalAlignment(Element.ALIGN_CENTER);
PdfPCell cell1_08 = new PdfPCell(new Paragraph(table[i][9], font));
cell1_08.setHorizontalAlignment(Element.ALIGN_RIGHT);
pdfPTable.addCell(cell1_01);
pdfPTable.addCell(cell1_02);
pdfPTable.addCell(cell1_03);
pdfPTable.addCell(cell1_04);
pdfPTable.addCell(cell1_05);
pdfPTable.addCell(cell1_06);
pdfPTable.addCell(cell1_07);
pdfPTable.addCell(cell1_08);
if(i != 0 && i % LIST_ROW == LIST_ROW-1) {
// 改頁
doc.newPage();
}
}
doc.add(pdfPTable);
} catch (DocumentException e1) {
JOptionPane.showMessageDialog(contentPane, “tableが、ありません。”,
“インフォメーション”, JOptionPane.INFORMATION_MESSAGE);
// TODO 自動生成された catch ブロック
e1.printStackTrace();
} catch (Exception e1) {
JOptionPane.showMessageDialog(contentPane, “pdfファイル作成できません。”,
“インフォメーション”, JOptionPane.INFORMATION_MESSAGE);
// TODO 自動生成された catch ブロック
e1.printStackTrace();
}
//文章オブジェクト クローズ
doc.close();
//PDFWriter クローズ
pdfwriter.close();
//JOptionPane.showMessageDialog(contentPane, “pdfファイル出力完了”,
// “インフォメーション”, JOptionPane.INFORMATION_MESSAGE);
}
/**
* 改ページ処理。
* このメソッドはページ作成終了時に呼び出される
*
* @param writer PDFライターオブジェクト
* @param doc ドキュメントオブジェクト
*/
@Override
public void onEndPage(PdfWriter pdfwriter, Document doc){
try {
//フォントの設定
Font font = null;
Font fontB =null;
Font fontU =null;
try {
font = new Font(BaseFont.createFont(“HeiseiKakuGo-W5”,
“UniJIS-UCS2-H”,BaseFont.NOT_EMBEDDED),9);
fontB = new Font(BaseFont.createFont(“HeiseiKakuGo-W5”,
“UniJIS-UCS2-H”,BaseFont.NOT_EMBEDDED),9, Font.BOLD);
fontU= new Font(BaseFont.createFont(“HeiseiKakuGo-W5”,
“UniJIS-UCS2-H”,BaseFont.NOT_EMBEDDED),9, Font.UNDERLINE);
} catch (DocumentException | IOException e2) {
// TODO 自動生成された catch ブロック
e2.printStackTrace();
}
// ————————-
// タイトルテーブル
// ————————-
float H_TABLE_COLUMN_WIDH[]= {1, 3.5f};
PdfPTable htable = new PdfPTable(H_TABLE_COLUMN_WIDH.length);
PdfPCell cell = null;
// テーブルの設定
htable.getDefaultCell().setPadding(0f);
htable.getDefaultCell().setLeading(16f, 0f);
// テーブル全体幅の設定
htable.setTotalWidth(doc.getPageSize().getWidth() – 80);
// テーブル列幅の設定
htable.setWidths(H_TABLE_COLUMN_WIDH);
// その他設定
htable.getDefaultCell().setBorder(0);
htable.setHorizontalAlignment(Element.ALIGN_CENTER);
htable.setLockedWidth(true);
// タイトル
cell = new PdfPCell(new Phrase(getTitle(pdfwriter, doc), fontB));
cell.setColspan(H_TABLE_COLUMN_WIDH.length);
cell.setHorizontalAlignment(Element.ALIGN_CENTER);
cell.setPaddingBottom(20f);
cell.setBorder(0);
htable.addCell(cell);
htable.writeSelectedRows(0, -1, 40, 570, pdfwriter.getDirectContent());
if (pdfwriter.getPageNumber() == 1) {
}
} catch (Throwable e) {
e.printStackTrace();
}
}
/**
* ヘッダタイトルを取得する。
*
* @param writer PDFライターオブジェクト
* @param doc ドキュメントオブジェクト
*/
private String getTitle(PdfWriter pdfwriter, Document doc) {
StringBuffer sb = new StringBuffer();
sb.append(“* 取引明細一覧表 *”);
sb.append(“(”);
sb.append(pdfwriter.getPageNumber());
sb.append(“/”);
sb.append(pageCount);
sb.append(“ページ)”);
return sb.toString();
}
}
}